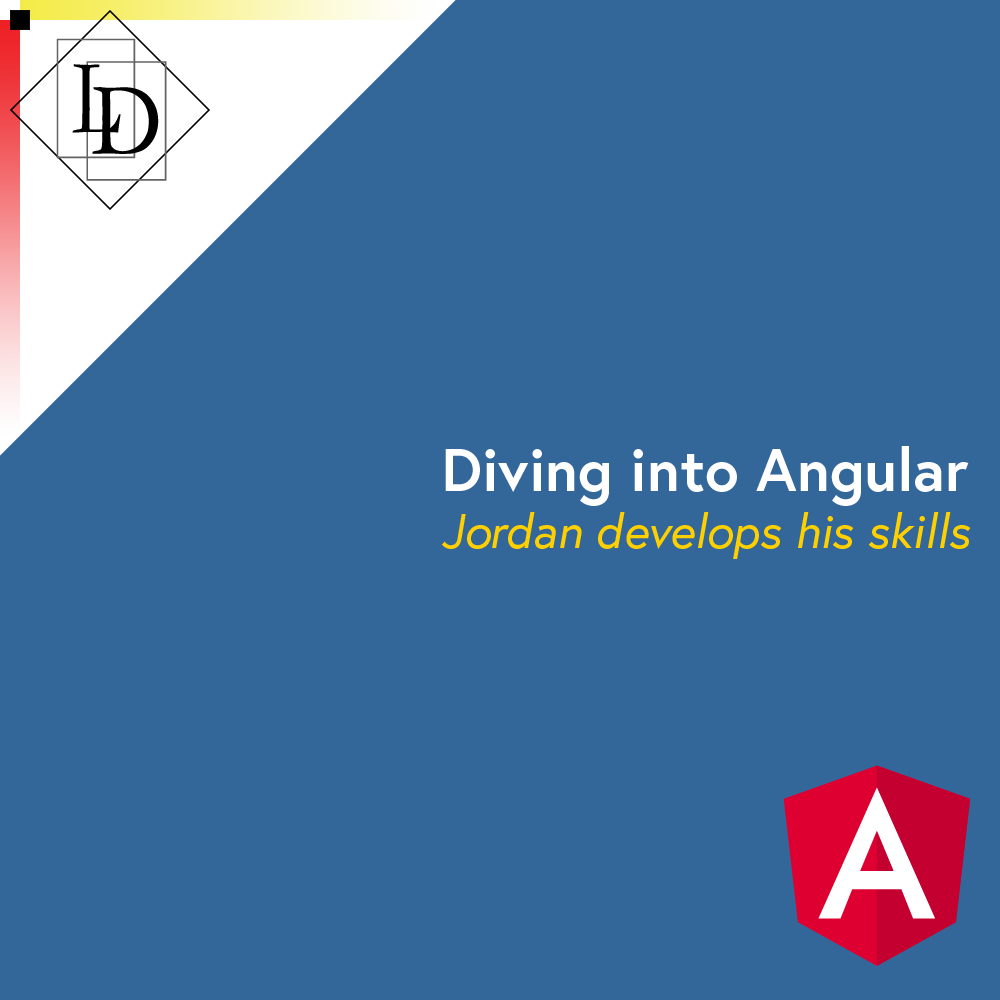
Diving into Angular, Jordan develops his skills
Angular is a web framework that combines HTML, CSS and Typescript. It is a compiled framework that dynamically recompiles segments as they are changed for fast live development. A web framework is a set of resources and tools that can be utilised to both build and manage websites, web services and web applications.In contrast a Library refers to a set of class definitions that performs defined operations. A library is designed for code reuse. If you wanted to replace a library you can easily swap it for another library without too much issue but a framework would require a rewrite of the entire code base. Another way to explain it would be that a Library extends the functionality of an application and cannot be used on it’s own while a framework provides the base functionality and can be used by itself.
Angular enables the easy incorporation of Typescript and JavaScript elements with HTML without the requirement for script tags. This lets us create special styling using calculated data that otherwise would be difficult to implement. Angular dynamically recompiles each segment during development. This enables quick website design and testing because you will see the changes in real-time getting quicker error reports to find and fix mistakes in the code. Not to be confused with the original Angular (now known as AngularJS), which was based off JavaScript. Angular 2 onward was completely rewritten from the ground up, with the use of Typescript, and functions in a completely different way to the original AngularJS. While Angular does incorporate the use of JavaScript it is through Typescript. Typescript is backwards compatible with JavaScript meaning all JavaScript is compatible with Typescript. However Typescript is not always compatible with JavaScript due to the new features it provides. Due to these differences the two versions of Angular are not compatible.
Using Angular effectively requires knowledge of two languages and a third that, while not vital for using Angular, is highly recommended. Angular combines HTML, Typescript (JavaScript) and CSS. CSS is just styling, while useful, does not inform how to build the page. An Angular application can be created without the use of CSS and hence while the knowledge is recommended, CSS is not something vital to using Angular. To use Angular you must first know how to use HTML and Typescript. HTML is vital for building any website and is the language of the internet. Since Angular is a website building tool you must therefore know how to write HTML code. Typescript is the second most important language to use Angular. Typescript is a super-set of the JavaScript language that adds optional static typing, classes and interfaces.
Originally I learned Angular by following the Angular tutorial on angular.io. While the tutorial is useful for a very basic understanding it is limited – only helping you learn how it works and how to create a very simple website but not much more. When I tried to implement it in my first project I found it slightly useful, but had to keep referring to other examples.
When it came to features I didn’t know, for the project I developed, I used a combination of trial and error as well as research. I’d apply the principles of programming (similarities found in most programming languages) to attempt to solve the problem. If that didn’t work then I would lookup solutions that did similar if not the same functionality I required. I then examined how it was performed and did it myself. When searching for solutions I generally found Stack Overflow and w3schools to be useful.
Currently, I am now learning Angular through a course on Udemy. The Udemy course is quite useful, the sequence of videos are well planned but not all of them are up to date and instead have notes that can make some segments confusing. The examples however are still clear and the instructor explains things in what I find to be an easy to understand way. This is because he writes and explains the code allowing me to also code along.
While I have not yet finished the course it has already taught me a lot of features I did not know during the original project. In that project all of the HTML was written in one file, but it is possible to separate a project into multiple files for each segment making it easier to read and modify.
Useful Utilities
Data binding and string interpolation
Angular provides a feature known as string interpolation which is a very powerful tool. String interpolation is where Angular takes a value from a defined variable and puts it into the HTML. String interpolation is encapsulated using double curly braces with the variable to be interpolated in the centre:
“{{ variable }}”
String interpolation can be used both in the text of an object and in the properties of HTML objects. The variable can be defined either in the Typescript for the page or in the HTML itself allowing for dynamic properties or text to be used rather than predefined static text.
Angular also provides Data binding. Data binding can be used to bind to properties, attribute and classes. To bind to a property you could use string interpolation, however string interpolation as the name states is only for strings. If a property requires a non-string value then you must use property binding. Property binding is done by enclosing the property in square brackets. Square brackets inform angular that you are targeting a DOM element. The following value is then a variable written in a string. For example:
<img [src]='itemImageUrl'>
The brackets tell angular to use the variable named itemImageUrl instead of using the string “itemImageUrl”. This allows us to create more dynamic properties. Data binding can be used in a few more places but I have not yet progressed enough in the course to cover it.
Dynamic Lists
Another example of the use of Angular was to create a dynamic list. In Angular, the structural directive *ngFor can be used to create a list that runs for each element in a defined range or list. The * in *ngFor tells Angular that we are using a structural directive, which is a special directive in Angular. “ng” Is used as a prefix to refer to Angular. When running Angular specific commands the ng prefix is usually used. This is done to separate an Angular command from any other type of command made by the user, environment or any external resources.
Taking a look at the documentation: a directive is a class “that add(s) additional behaviour [to] Angular applications”. Angular has three types of directives, these being Components, Attribute and Structural. Components are directives with a template. Attribute directives are directives that changes the appearance or behaviour of elements, components or even other directives. Finally Structural directives are directives that interact with the DOM layout, they can add or remove DOM elements.
To give an example, The *ngFor structural directive can be written as such:
<li *ngFor=”Let item of list”> {{item}} </li>
Which Angular then translates as:
<ng-template ngFor let-item [ngForOf]=”list”>
<li> </li>
</ng-template>
A template is essentially HTML content that is only rendered when instructed to do so and can be used by structural directives as many times as required. Meaning by using *ngFor we use the template to load a list element for each item.
Usually, to create a list we would need to know each list item and make a list element with the data for every item. However, by using angular structural directives we only need to create one list element and angular will do the rest for us.
I hope to master Angular to be able to greatly improve website creation. Angular is a very useful tool and I’ve been able to create with basic knowledge a website that was already more advanced than the HTML website I created in year 8 for the National History Challenge. Angular would have made a nicer website for the challenge because it is a powerful tool. However, while using Angular would be a good choice if I were to re-do that project today, it requires the underlying knowledge of HTML and Typescript (that I didn’t have in year 8).
Currently I am about a quarter through the Udemy Angular Course and I plan to continue it until it is completed.